
Step 3 of 7: MPI Sample
Programs
The following example has been provided as a
reference for beginning MPI programmers. Reading through and
executing these programs should be helpful as you begin to
write your own code. Note that you can download the code by
clicking on this link.
#include "mpi.h"
#include <stdio.h>
#include <math.h>
#define MAXSIZE 1000
int add(int *A, int low, int high)
{
int res, i;
for(i=low; i<high; i++)
res += A[i];
return(res);
}
void main(argc,argv)
int argc;
char *argv[];
{
int done = 0, n, myid, numprocs;
int data[MAXSIZE], i, low, high, myres, res;
char fn[255];
char *fp;
MPI_Init(&argc,&argv);
MPI_Comm_size(MPI_COMM_WORLD,&numprocs);
MPI_Comm_rank(MPI_COMM_WORLD,&myid);
n = 0;
while (!done)
{
if (myid == 0)
{
if (n==0) n=100; else n=0;
strcpy(fn,getenv("HOME"));
strcat(fn,"/MPI/rand_data.txt");
/* Open Input File and Initialize Data */
if ((fp = fopen(fn,"r")) == NULL)
{
printf("Can't open the input file: %s\n\n", fn);
exit(1);
}
for(i=0; i<MAXSIZE; i++)
fscanf(fp,"%d", &data[i]);
}
MPI_Bcast(&n, 1, MPI_INT, 0, MPI_COMM_WORLD);
MPI_Bcast(data, MAXSIZE, MPI_INT, 0, MPI_COMM_WORLD);
if (n == 0)
done = 1;
else
{
low = myid * 100;
high = low + 100;
myres = add(data, low, high);
printf("I got %d from %d\n", myres, myid);
MPI_Reduce(&myres, &res, 1, MPI_INT, MPI_SUM, 0, MPI_COMM_WORLD);
if (myid == 0)
printf("The sum is %d.\n", res);
}
}
MPI_Finalize();
}
|
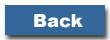
|