
Step 5 of 9: Sample
Programs
The following are sample programs which are to be saved
in the src directory and then compiled. Compilation and
execution of these programs are explained in later
steps.
In the following sample programs, the master will send
the message "Hello World!" to the slaves. Each
slave program will print the message "Hello
World!" it received from the master program and print
it.
Master.C
#include
<stdio.h>
#include “pvm3.h”
#define NTASKS 6
#define HELLO_MSGTYPE 1
char helloworld[13] = "HELLO
WORLD!";
main() {
int mytid,
tids[NTASKS],
i,
msgtype,
rc,
bufid;
/* char helloworld[13] = "HELLO WORLD!";
*/
for (i=0; i<NTASKS; i++)
tids[i] = 0;
} |
Slave.C
#include <stdio.h>
#include "pvm3.h"
#define HELLO_MSGTYPE 1
main() {
int mytid,
msgtype,
rc;
char helloworld[13];
mytid = pvm_mytid();
msgtype = HELLO_MSGTYPE;
rc = pvm_recv(-1, msgtype);
rc = pvm_upkstr(helloworld);
printf(" ***Reply from spawned process: %s : \n",helloworld);
rc = pvm_exit();
} |
NOTE: To compile these programs, you have to
use Makefile.aimk. The next step describes the
Makefile.
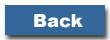
|