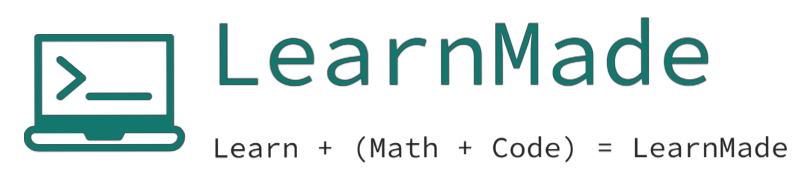
If you ask someone what their first program was, odds are good they will tell you "Hello, World!"
"Hello, World!" is a very simple program that outputs "Hello, World!" to the console. The program consists of a class (can be named whatever, in our example it is named Main) that contains a main method (sometimes called the driver) and within it a single print statement, as follows:
public class Main
{
public static void main(String[] args)
{
System.out.println("Hello, World!");
}
}
*Note Java is case-sensitive, so system.out.println(); would result in an error
Notice the semicolon? Java is compiled, so it requires a semicolon after each instruction in order for the compiler to identify where an instruction ends. The compiler is a program that compiles source code (high level) down to bytecode (middle level), which is then interpreted and executed by the Java Virtual Machine (JVM).
Activity 1) Fill in the code
Main
{
public static void (String[] args)
{
.out.println("LearnMade rocks!");
}
}
Your answer | Correct answer | ||
---|---|---|---|
1. | class | ||
2. | main | ||
3. | System |
Grouping: looking back at our example:
public class Main
{
public static void main(String[] args)
{
System.out.println("Hello, World!");
}
}
The class, Main, contains the main method, which contains the code to print "Hello, World!" This concept of containment, formally called encapsulation when relating to a class containing fields, variables, methods, and various other properties (covered later), is the essence of a group containing something.
Anything inside of set braces {...} is a member of the group. A group can be something like a class, method, conditional statement, or loop, where everything within its set braces belongs to it.
Naming Conventions:
*We will talk about classes, keywords, variables, and methods later
camelCase is a naming convention where the first character of the first word is lowercase, and then every successive word's first character is Uppercase; example: lowerUpper
Activity 2) Identify the correct naming
Your answer | Correct answer | ||
---|---|---|---|
1a. | true | ||
1b. | false | ||
1c. | true | ||
1d. | false | ||
1e. | true |
You might be wondering what int myInt; and double d; are, which leads us to the next topic: variables and data types.