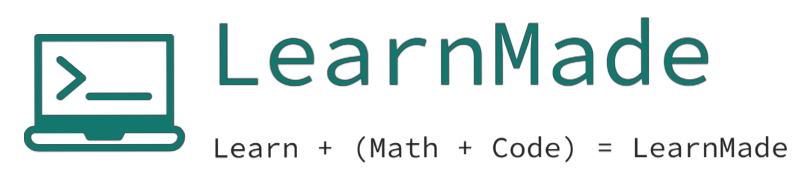
If you ask someone what their first program was, odds are good they will tell you "Hello, World!"
"Hello, World!" is a very simple program that outputs "Hello, World!" to the console. Unlike in Java, this program simply consists of a single print statement, as follows:
print("Hello, World!")
*Note Python is case-sensitive, so Print() would result in an error
Notice there is no semicolon? Unlike most languages (Java, C++, etc.), Python does not require a semicolon after each instruction, because it is interpreted. Source code (high level) is interpreted and executed (bytecode, middle level) by the Python Virtual Machine/Environment (PVM/VENV).
Activity 1) Print "Hello, how are you?"
Your answer | Correct answer | ||
---|---|---|---|
1. | print("Hello, how are you?") |
Grouping: consider the example:
def message(word):
message = "Your message: " + word
print(message)
message("Consistency is key!")
Above is a simple program that defines a function and calls it. The function takes an argument, modifies it, and prints it;
the function call, message("Consistency is key!"), will output "Your message: Consistency is key!" The point of including this code snippet is not
to show what it does, rather to illustrate the manner in which the function, message, contains code.
*Don't worry about the details of what a function, argument, and function call are yet, it will all be covered in later sections
This concept of containment, formally called encapsulation when relating to a class containing variables, methods, and other properties (covered later), is the essence of a group containing something. Unlike most languages (Java, C++, etc.) Python does not use set braces {...} to denote groups.
In Python, a group starts with a colon : and everything within it must be consistently indented (by spaces or tabs).
A group can be something like a class, method, conditional statement, or loop, where everything that's consistently indented after a colon : is a member of the group.
Once the indentation is broken (to meet the previous level of indentation), the group is considered concluded,
and the breaking element is not considered part of the group
(in the example, line 4 is not part of the function, message, because it went back a level).
*Note if the indentation is broken by going in more, a syntax error will be thrown
Naming Conventions:
*We will talk about classes, keywords, variables, and methods later
camelCase is a naming convention where the first character of the first word is lowercase, and then every successive word's first character is Uppercase; example: lowerUpper
Activity 2) Where does it break?
Consider the code:
*Line numbers are denoted in the code by #x
#0 def message(word):
#1 message = "Your message: " + word
#2 print(message)
#3 message("Consistency is key!")
#4
#5 def error(x,y):
#6 x += 2
#7 y += 2
#8 return x + y
#9 error(6, 28)
1. What is the last line of the function, message?
2. What line throws a syntax error?
Your answer | Correct answer | ||
---|---|---|---|
1. | Line 2 | ||
2. | Line 7 |